How to Create an ERC-20 Token in 10 Minutes
Creating an ERC-20 token on the Ethereum blockchain is a straightforward process that requires minimal coding knowledge. With just a few steps, you can deploy your own token in under 10 minutes. In this guide, we’ll walk through the entire process, from setting up your development environment to deploying your ERC-20 token on Ethereum.
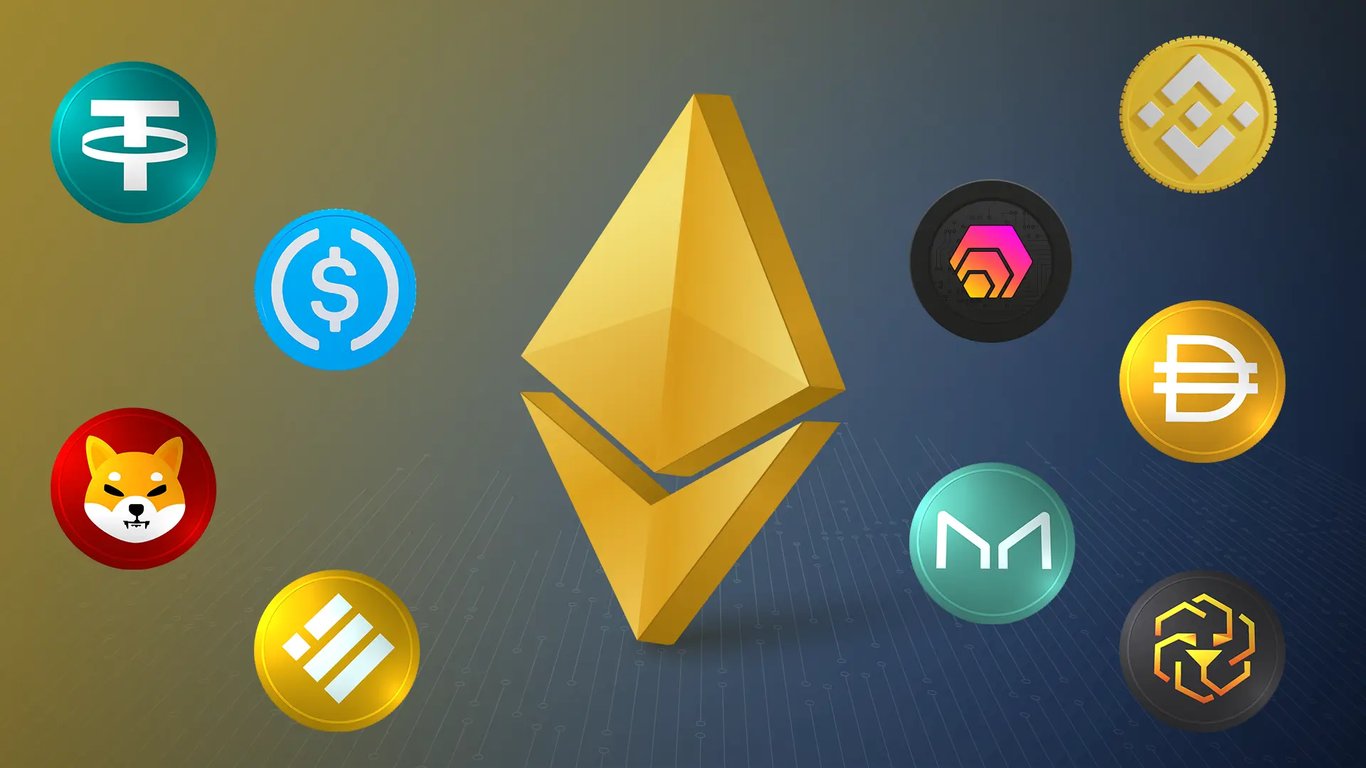
What is an ERC-20 Token?
ERC-20 is the most widely used token development standard on Ethereum. It ensures compatibility with wallets, exchanges, and decentralized applications (dApps). ERC-20 tokens are used for various purposes, such as governance, payments, rewards, and utility tokens in blockchain ecosystems.
Prerequisites
Before we begin, ensure you have the following:
MetaMask Wallet – To interact with the Ethereum blockchain.
Ether (ETH) – To pay for gas fees on the Ethereum network.
Remix IDE – An online Solidity compiler for smart contracts.
Solidity Programming Knowledge – Basic understanding is helpful but not mandatory.
Step-by-Step Guide to Creating an ERC-20 Token
Step 1: Setting Up Remix IDE
Remix is an online Solidity compiler that allows you to write and deploy smart contracts easily.
Visit Remix IDE in your web browser.
Create a new file and name it MyToken.sol.
Step 2: Writing the ERC-20 Smart Contract
Copy and paste the following Solidity code into the MyToken.sol file:
solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyToken is ERC20, Ownable {
constructor() ERC20("MyToken", "MTK") {
_mint(msg.sender, 1000000 * 10 ** decimals());
}
}
Explanation of the Code:
ERC-20 Standard: We import the ERC-20 implementation from OpenZeppelin, which ensures security and efficiency.
Token Name & Symbol: The constructor sets the token name to “MyToken” and the symbol to “MTK.”
Minting Tokens: The _mint function generates 1,000,000 MTK and assigns them to the contract owner.
Ownable Contract: This allows only the contract owner to manage certain functions.
Step 3: Compiling the Smart Contract
Click on the Solidity Compiler tab in Remix.
Select Solidity version 0.8.x (same as in the contract).
Click Compile MyToken.sol.
If successful, you will see a green checkmark indicating the contract is compiled.
Step 4: Deploying the Smart Contract
Go to the Deploy & Run Transactions tab.
Select Injected Web3 as the environment (connects to MetaMask).
Choose the Ethereum testnet (e.g., Goerli, Sepolia) or mainnet in MetaMask.
Click Deploy and confirm the transaction in MetaMask.
Once deployed, you will receive the contract address, which represents your ERC-20 token on the Ethereum blockchain.
Step 5: Verifying and Using Your Token
Copy the deployed contract address.
Open Meta Mask and go to the Tokens section.
Click Import Tokens and paste your contract address.
Your token should now be visible in MetaMask!
Conclusion
Congratulations! You have successfully created and deployed an ERC-20 token in under 10 minutes. This token can now be used for various purposes, including fundraising, staking, and governance in decentralized applications.
For further enhancements, you can add features like burning, pausing, or vesting schedules to your token. If you want to launch your token on a large scale, consider conducting an audit and deploying it on the Ethereum mainnet.
Like my work? Don't forget to support and clap, let me know that you are with me on the road of creation. Keep this enthusiasm together!